In Python, iterating over a dictionary is a crucial skill for developers to be able to access and manipulate key-value pairs efficiently. It allows them to perform different operations, including updating information, extracting values, and applying a particular logic to every key-value pair.
Moreover, developers must know how to iterate over a dictionary in Python to handle and process data while interacting effectively with the contents of a dictionary. This blog discusses different ways to iterate through a dictionary in Python, explaining each method with an example.
Python Dictionaries
Dictionaries are useful data structures in Python that store items in key-value pairs. Keys on dictionaries are hashable, so their values can’t be changed. Also, there can’t be duplicate keys in a dictionary. We can access values stored in a dictionary using keys. Many aspects of Python are built around dictionaries. Classes, modules, objects, locals(), and globals() show how deeply dictionaries are wired into the implementation of Python.
The keys in a dictionary are like a set. They are hashable (so we can’t use mutable objects as keys) and unique objects. Values in a dictionary can be of any data type, be it hashable or non-hashable.
Key points to remember about Python dictionaries
- Dictionaries store items in key-value pairs. They map keys to values and store them in a collection.
- Dictionaries are indexed by keys, so we can access values stored in them using their associated keys.
- Keys in dictionaries are hashable, so they must have a hash value that can never change during a key’s lifetime.
Also read: 10+ Best Python Books For Beginners and Experts (2025 List)
How to Iterate Over a Dictionary in Python
Iterating through a dictionary refers to going through each key-value pair one by one, accessing a dictionary, and traversing every key-value present. It is crucial to use a Python dictionary properly. In Python, iterating over a dictionary is possible through various ways that we have discussed below:
- Iterate through Python dictionary using build.keys()
- Iterate key-value pair using items()
- Iterate through all values using .values()
- Looping through a dictionary using for loop
- Access a key in Python using zip()
- Access key using map() and dict.get
- Access key Using Unpacking of dictionary
1. Iterate through Python dictionary using build.keys()
In Python, we can use the built-in keys() method to access the keys of a dictionary. It is a simple method to iterate over and manipulate the structure of a dictionary and returns a view object displaying a list of all the keys in the dictionary. The keys() method allows us to efficiently traverse the keys of a dictionary, making it suitable for tasks that involve working with the keys themselves.
Example
my_dict = {'name': 'Harshit', 'age': 25, 'city': 'Jodhpur'}
for key in my_dict.keys():
print(key)
Output
name
age
city
2. Iterate key-value pair using items()
Using the built-in items() method in Python, we can access keys and items at the same time, so we can effectively manipulate the entire structure of the dictionary. This powerful tool allows us to iterate through key-value pairs and returns the view object containing a key-value pair as tuples.
Using items() provides a concise way to navigate through the entire dictionary, extracting keys and values as needed. It is a useful method for tasks that require simultaneous access to both components of every key-value pair.
Example
my_dict = {'name': 'Harshit', 'age': 25, 'city': 'Jodhpur'}
for key, value in my_dict.items():
print(f"{key}: {value}")
Output:
name: Harshit
age: 25
city: Jodhpur
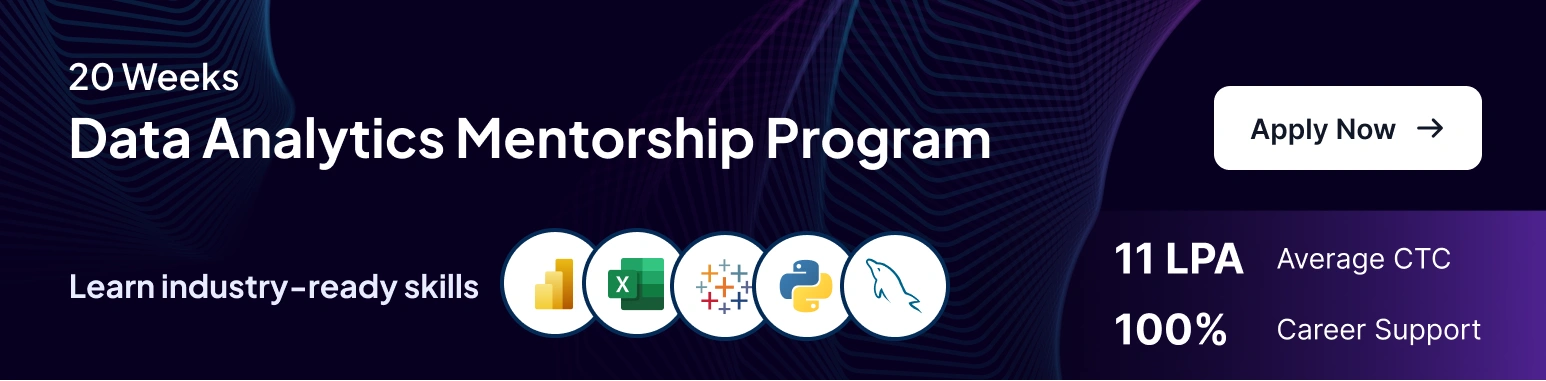
3. Iterate through all values using .values()
The values() method is another straightforward way to efficiently iterate through all values of a dictionary in Python. Hence, it is useful for tasks that involve working with values directly instead of keys associated with them.
It returns a view object with all the values present in the dictionary, so we can access and manipulate data without explicitly dealing with keys. The values() method provides the flexibility of manipulating a dictionary in Python. To use the values() method to iterate over all values of a dictionary, employ the for loop, accessing every value sequentially. You can process or display every individual value in the dictionary without explicitly referencing the associated keys.
Example
my_dict = {'name': 'Harshit', 'age': 25, 'city': 'Jodhpur'}
for value in my_dict.values():
print(value)
Output
Harshit
25
Jodhpur
4. Looping through a dictionary using for loop
Using a for loop is also a fundamental and versatile way to iterate through a dictionary. This method allows us to easily access keys in a dictionary without using the keys() method and directly iterate over the dictionary using a for loop.
The for loop iterates automatically over the keys, so we can access every key without explicitly needing the method call. We can manipulate data stored within efficiently and seamlessly. This is particularly useful for working with the keys of a dictionary.
Example
my_dict = {'name': 'Harshit', 'age': 25, 'city': 'Jodhpur'}
for key in my_dict:
print(key)
Output:
name
age
city
5. Access a key in Python using zip()
The zip() function in Python allows us to access the keys of a dictionary by iterating over a tuple of keys and values of a dictionary. Using this method in conjunction with the keys of a dictionary, we can create pairs of keys and their corresponding values.
We can iterate through keys without directly accessing the dictionary. Hence, it is useful when we work with key-value pairs as tuples. It improves the iterating flexibility through dictionary keys in an expressive and readable manner.
Example
my_dict = {'name': 'Harshit', 'age': 25, 'city': 'Jodhpur'}
keys = list(my_dict.keys())
values = list(my_dict.values())
for key, value in zip(keys, values):
print(f"{key}: {value}")
Output:
name: Harshit
age: 25
city: Jodhpur
Also read: 25+ Python Project Ideas (Beginners to Experienced)
6. Access key using map() and dict.get
Python also offers the map() function with dict.get() to access keys in a dictionary. The combination of map() and dict.get() is a concise and efficient approach to applying a function to each key, returning a map object of corresponding values. It returns a new iterable containing the results.
This method allows direct iteration over dictionary keys and efficiently obtains their values concisely. It improves the flexibility of working with dictionaries.
Example
my_dict = {'name': 'Harshit', 'age': 25, 'city': 'Jodhpur'}
keys = my_dict.keys()
values = map(my_dict.get, keys)
for value in values:
print(value)
Output:
Harshit
25
Jodhpur
7. Access key using unpacking of dictionary
Unpacking is a simple and efficient way to access and iterate through dictionary keys without calling any method explicitly. This process involves extracting keys directly from a dictionary and then working with each of them individually. Unpacking is a readable and concise method to access keys and can come in handy when we want a list of keys without any additional methods or functions.
When we work with Python dictionaries, unpacking improves the code clarity and expressiveness. We use asterisk (*) to unpack the keys into a list or another iterable and access them through unpacking of a dictionary.
Example
my_dict = {'name': 'Harshit', 'age': 25, 'city': 'Jodhpur'}
for key in *my_dict,:
print(key)
Output:
name
age
city
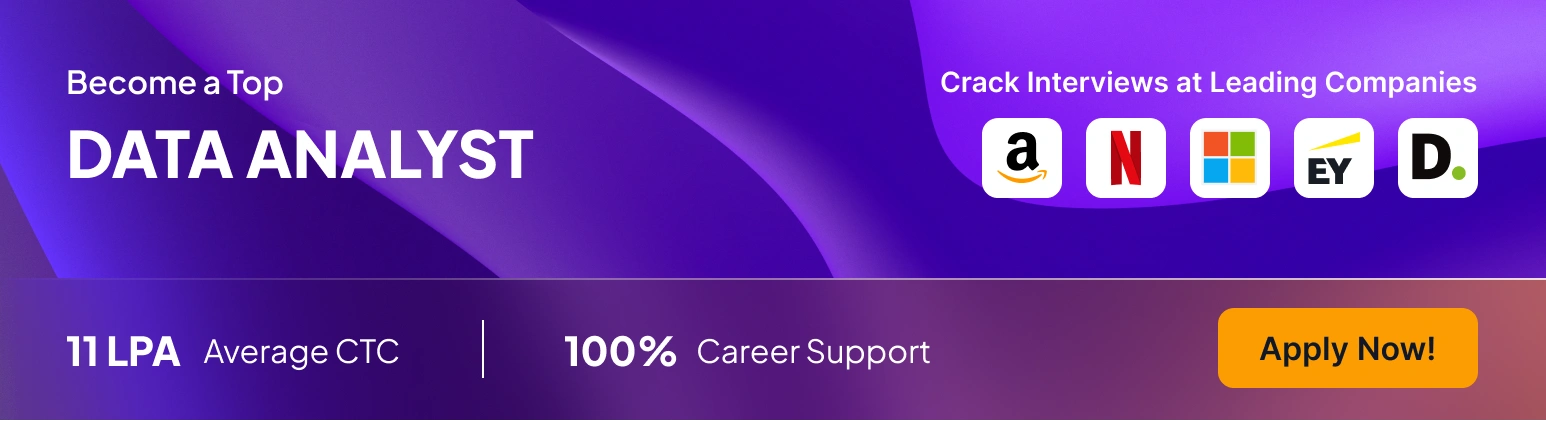
FAQs- Iterating Over a Dictionary in Python
In Python, collections and itertools standard-library modules offer the ChainMap class and chain() function, respectively, that we can use to combine multiple dictionaries and iterate through them. However, these tools have a few basic differences. In the case of duplicate keys in input dictionaries, ChainMap provides only access to the first instance of a key, whereas chain() allows access to all the instances of repeated keys.
We can also use the unpacking operator (**) to combine multiple dictionaries and iterate through the resulting dictionary. When the input dictionaries have duplicate keys, only the last instance of the repeated key will prevail.
We can iterate over an item of a dictionary using a for loop. This will return key-value pairs.
We use the built-in sorted() function to iterate through a dictionary in a specific order. If fed with the result of .items(), the function will return a list of key-value tuples that we can traverse in a loop. We can also use the reversed() function to iterate through a dictionary in reverse order.
To change or update the values of a dictionary, we can use a loop because dictionaries are mutable, so we can change their values easily. However, to remove key-value pairs from a dictionary while looping through it, use a copy for iteration and remove the values from the original dictionary. The copy() method allows you to create a shallow copy of a dictionary. The popitem() method is used with the while loop to remove consecutive items from a dictionary without making its copy.
We use the values() method in Python to get an iterable of values for a dictionary.
We use a for loop to iterate over the values of a dictionary in Python
Conclusion
Knowing how to iterate through a dictionary in Python is crucial as it enables you to work with data seamlessly. Also, it is a vital skill if you want to access the keys and values of a dictionary. Hence, whether you are a beginner or an experienced Python programmer, learning how to iterate through a dictionary will make working with data hassle-free and efficient. You will have a powerful tool to manipulate and navigate information effectively in Python programs.
In this blog, we explored different methods to iterate over a dictionary, such as values(), keys(), items(), map(), zip(), loops, unpacking, etc. There are diverse ways to handle dictionaries, and you can pick the one that suits your goals and needs.
Read more blogs