Python arrays and lists are data structures used to store multiple elements. We use indexing of elements for accessing, iterating, and slicing. Although both serve the same purposes, they differ in their features, which affect how data is accessed and managed while programming.
Python developers must understand the difference between arrays and lists to write efficient code and manipulate data effectively.
This blog will discuss list vs array in detail, including their usage, properties, and more.
What Are Lists?
A list in Python is an in-built data structure to hold a collection of elements of different data types. It stores several items into a single variable, containing data that can be numerical, character logical values, etc.
Lists are dynamic, so we can change their size during program execution. Hence, lists can be used to perform tasks that involve adding or removing elements. This ordered collection supports negative indexing and can be created using [], which contains data values. We can merge and copy elements of a list using the built-in functions in Python.
Through lists, developers have a convenient interface to manage and organize data, which enables efficient operations, including inserting, appending, and deleting elements. As a list can dynamically adjust its size, it can be a powerful tool to handle different amounts of data in a program.
Features of Python Lists
The following are the key characteristics of lists in Python:
- They are the in-built data structures.
- They can contain any arbitrary object.
- They can be nested to arbitrary depth.
- List lements are enclosed within square brackets [] and separated using commas.
- They are an ordered collection of elements of different types.
- They are dynamic and mutable.
- They can nest lists with other data structures, such as dictionaries, tuples, and lists.
- We can access elements in a list using indexing.
Example
# Creating a list with different data types
my_list = [10, "Python", 3.14, True]
print("Original list:", my_list)
# Accessing elements using positive and negative indexing
print("First element:", my_list[0]) # Positive index
print("Last element:", my_list[-1]) # Negative index
# Modifying an element (lists are mutable)
my_list[1] = "Programming"
print("Modified list:", my_list)
# Adding elements to the list
my_list.append("New Element") # Appending at the end
print("After appending:", my_list)
# Inserting an element at a specific position
my_list.insert(2, "Inserted Element")
print("After inserting:", my_list)
# Removing elements
my_list.remove(3.14) # Removes specific value
print("After removing an element:", my_list)
# Copying the list
copied_list = my_list.copy()
print("Copied list:", copied_list)
# Merging two lists
new_list = [1, 2, 3]
merged_list = my_list + new_list
print("Merged list:", merged_list)
# Nested lists
nested_list = [my_list, new_list]
print("Nested list:", nested_list)
# Accessing elements in a nested list
print("First element of the nested list:", nested_list[0][1])
Output
Original list: [10, 'Python', 3.14, True]
First element: 10
Last element: True
Modified list: [10, 'Programming', 3.14, True]
After appending: [10, 'Programming', 3.14, True, 'New Element']
After inserting: [10, 'Programming', 'Inserted Element', 3.14, True, 'New Element']
After removing an element: [10, 'Programming', 'Inserted Element', True, 'New Element']
Copied list: [10, 'Programming', 'Inserted Element', True, 'New Element']
Merged list: [10, 'Programming', 'Inserted Element', True, 'New Element', 1, 2, 3]
Nested list: [[10, 'Programming', 'Inserted Element', True, 'New Element'], [1, 2, 3]]
First element of the nested list: Programming
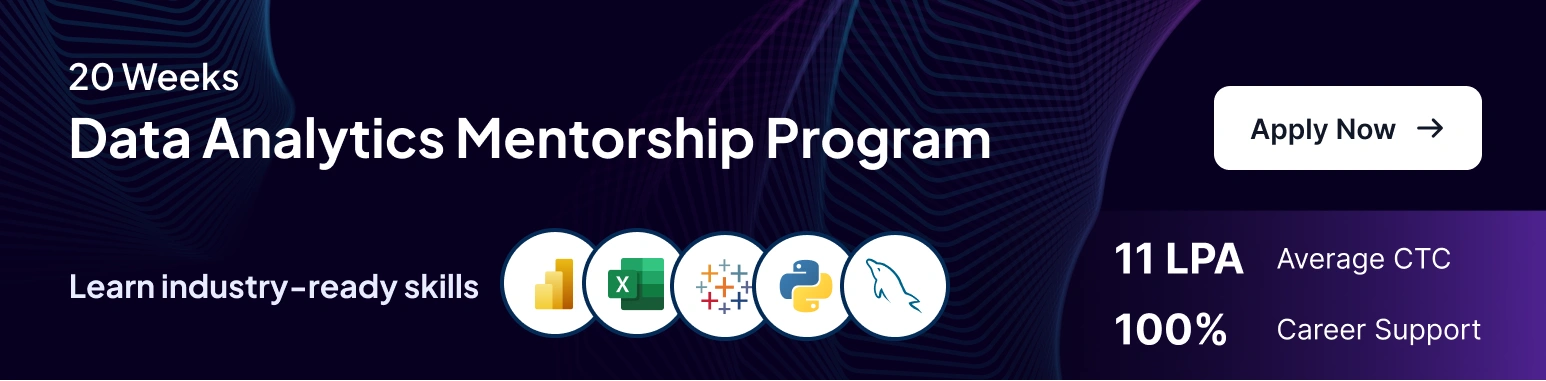
What Are Arrays?
An array is a collection of homogeneous elements, which means it contains the elements of the similar type in a contiguous memory block. The size of an array is fixed, and we can identify each array by an index that represents its position. It can store more than one value at a time. The cost of inserting and deleting elements is higher in an array than a list, but indexing is faster in an array because of contiguous memory allocation.
Arrays provide quick and direct access to their elements through indices. Also, they have a systematic way of organizing and managing data, so we can retrieve, change, and manipulate information efficiently. We can import the array using an array or NumPy module. Arrays are commonly used in programming for their effectiveness and simplicity in handling ordered data sets.
The main difference between list and array is that the former can store multiple data types at a time, whereas the latter can store only one data type.
Features of Python Arrays
Here are the primary features of arrays in Python:
- These in-built data structures are readily available in Python.
- Arrays are not the default data structures in Python.
- We can store only the same type of elements in an array.
- If we store different types of elements in an array, it will throw an error.
- This derived data type contains different primitive data types, such as float, int, and char.
- We can use only constants and literal values to create array elements.
- The base address of an array is stored in the array name, which serves as a pointer to the memory block in which we store elements.
- We can import an array using the array and NumPy module.
- We use the primary memory to store array elements in contiguous blocks.
Example
import array as arr
# Creating an array of integers
numbers = arr.array('i', [1, 2, 3, 4, 5])
print("Original array:", numbers)
# Accessing elements using indexing
print("First element:", numbers[0])
print("Last element:", numbers[-1])
# Modifying an element (arrays are mutable)
numbers[2] = 10
print("Array after modification:", numbers)
# Adding elements to the array
numbers.append(6) # Adds an element to the end
print("Array after appending:", numbers)
# Inserting an element at a specific position
numbers.insert(1, 15)
print("Array after inserting:", numbers)
# Removing elements
numbers.remove(4) # Removes the first occurrence of value 4
print("Array after removing an element:", numbers)
# Accessing array elements using a loop
print("Array elements:")
for element in numbers:
print(element)
Output
Original array: array('i', [1, 2, 3, 4, 5])
First element: 1
Last element: 5
Array after modification: array('i', [1, 2, 10, 4, 5])
Array after appending: array('i', [1, 2, 10, 4, 5, 6])
Array after inserting: array('i', [1, 15, 2, 10, 4, 5, 6])
Array after removing an element: array('i', [1, 15, 2, 10, 5, 6])
Array elements:
1
15
2
10
5
6
Also read: 25+ Python Project Ideas (Beginners to Experienced)
Operations Difference in Lists and Arrays
Accessing elements in a Python array is fast because they are in a contiguous block. However, inserting and deleting elements shift the elements from their position linearly, which increases the cost.
On the other hand, accessing elements in a Python list is similar to that in an array because a list is a dynamic array. Deleting or inserting elements at the beginning or in the middle of a list is not so efficient as it can shift all subsequent elements.
Difference Between Arrays and Lists in Python
Let’s discuss array vs list in Python based on various parameters:
Feature | Arrays | List |
Data Type | All elements in an array are of the same data type, i.e., homogeneous. | All elements in a list are heterogeneous, which means different data types. |
Printing | We can print the array elements by creating a specific loop. | We can print a list without explicitly creating a loop. |
Flexibility | An array is less flexible because modifying data or changing its size is difficult. | A list is highly flexible as we can easily modify data and add or remove elements. |
Memory Allocation | We can allocate the memory of an entire array during initialization. | In lists, memory is allocated dynamically as elements are added or removed. |
Types | In an array, the elements in a collection are of the same data type. | A list is a collection of different types of elements. |
Imports | We can import arrays using an array or NumPy. | We don’t need to import lists as they are in-built data structures. |
Numerical Operations | In arrays, we use specialized functions for numerical operations. | Lists provide built-in methods and functionalities. |
Access Time | Arrays have constant-time access to elements through indexing. | Lists have slightly variable-time access due to dynamic resizing. |
Size | Arrays have the same size as the array for nesting. | Variable-size nesting is possible. |
Broadcasting | Broadcasting and element-wise operations are possible. | No support for broadcasting efficiently. |
Memory Efficiency | If size is larger than required, it may cause memory wastage. | As memory is allocated dynamically, it is more memory-efficient. |
Arithmetic Operations | Direct application of arithmetic operations. | Direct application of arithmetic operations is not possible. |
Numerical Computations | Optimized for numerical computations, making it faster. | Slower for numerical computations. |
Memory | Consumes less memory than a list. | Consumes more memory. |
Syntax For Creation | Uses NumPy.array and .array function. | Uses square brackets [ ] |
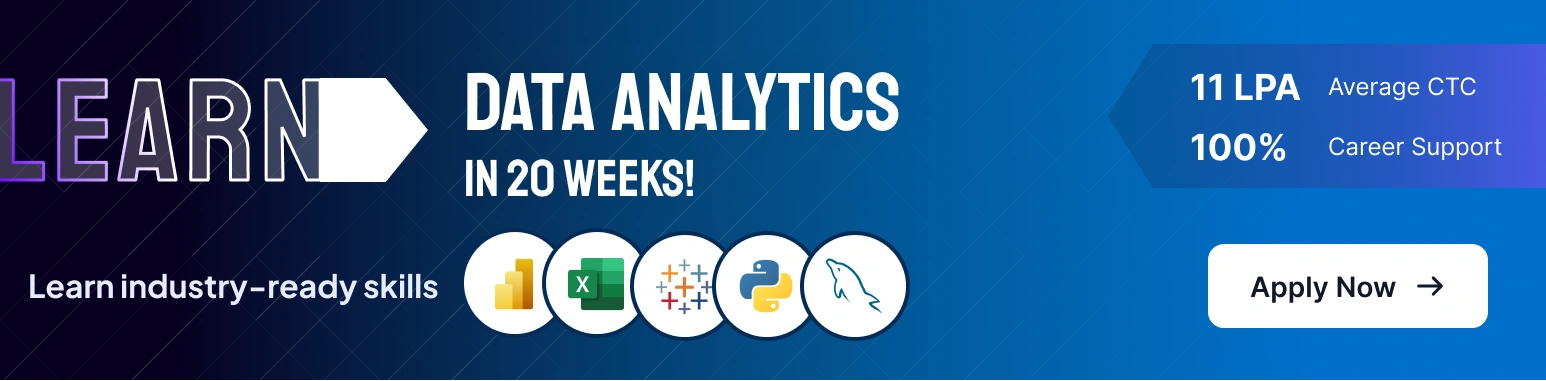
FAQs- Difference Between a List and an Array
In Python, arrays are comparatively more memory efficient than lists. They can store elements of the same data type, but lists store elements of different data types.
Python doesn’t have ArrayList as it is a data structure in Java. it is a resizable array-like structure. The closest equivalent to ArrayList in Python is a list that refers to an ordered collection of elements. A list can contain duplicate elements. A set is an unordered collection of unique elements that automatically removes a duplicate element. It doesn’t support operations that depend on a sequence.
Remove- It removes the first occurrence of a specific value from a list without returning the removed element. If the given value is not found, it raises a ValueError.
Syntax
my_list = [4, 5, 4, 7]
my_list.remove(4) # Removes the first ‘4’
Pop- It removes an element at a specific position or index and returns it. If the index is not mentioned, it removes and returns the last item given in the list. If the given index is out of range, it raises an IndexError.
Syntax
my_list = [1, 2, 3]
removed_element = my_list.pop(1) # Removes and returns ‘2’
List- It is an ordered collection of different types of elements. It is versatile and can handle a sequence of elements that must be ordered and changed.
Array- It is used to store homogeneous data efficiently in a compact way and can be imported using the array module.
Dictionary- It is an unordered collection of key-value pairs that is optimized for retrieving data. We can access elements using a unique key. Dictionaries are best for large datasets that require fast insertion, lookup, and deletion of elements.
Conclusion
We hope that you get to learn new and insightful information through this blog. As a Python developer, you must be able to tell the difference between array and list in Python. You must know the purpose they serve and their uses. A list groups elements of different data types in Python, whereas an array is the collection of the same data type. To learn about arrays, lists, and other Python concepts, join our Python course, which aims to hone your skills and transform you into a proficient Python programmer.